Create wallet button
For the best onboarding experience, we recommend adding a highly visible 'Create' or 'Create Wallet' button to your app's homepage. Adding this button streamlines the onboarding experience for new users and gets them ready to use your app in a few seconds.
Here is an example of how the button looks:
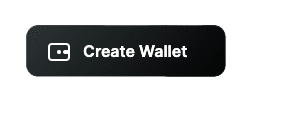
Implementation with Wagmi#
To make these instructions concrete, we have created a sample ecosystem wallet called Rapidfire ID. To interact with it, you can find its SDK in the NPM package directory: @rapidfire/id.
You can check out the GitHub repository for Rapidfire Wallet to learn how to create your own wallet.
Using Wagmi offers a balanced approach that combines flexibility with developer convenience. By integrating the ecosystem wallet with Wagmi, you get access to a comprehensive set of React hooks that handle common wallet operations, state management, and chain interactions. This approach requires both @rapidfire/id
and Wagmi
dependencies, along with React Query, but provides a more structured development experience. While you'll still need to build your own UI components, Wagmi's hooks significantly reduce the complexity of handling wallet connections, transactions, and chain switching.
Configure Wagmi
First, set up your Wagmi configuration with the required chain and connector settings.
Create the Wallet Logo Component
Create a reusable wallet logo component that will be used in the button.
Implement the Create Wallet Button
Create the main button component using Wagmi's useConnect hook.
For more detail, view the useConnect
documentation.
Upon successful connection, account information can be accessed via data returned from useConnect
, or via useAccount
.
Implementation with EIP-1193 Provider#
The EIP-1193 Provider approach gives you the most granular control over your ecosystem wallet integration by directly using the SDK's Ethereum provider. This method requires only the @rapidfire/id
package and allows you to build everything from scratch. You'll handle all wallet interactions through the standard EIP-1193
provider interface, giving you complete flexibility in implementing wallet creation, connection flows, and transaction handling. While this approach requires more custom development work, it's ideal for applications that need specialized wallet interactions or want to minimize dependencies.
To make these instructions concrete, we have created a sample ecosystem wallet called Rapidfire ID. To interact with it, you can find its SDK in the NPM package directory: @rapidfire/id.
You can check out the GitHub repository for Rapidfire Wallet to learn how to create your own wallet.
Initialize the SDK
Create a new SDK instance with your application configuration.
Set up the Provider
Configure the Ethereum provider with chain and policy settings.
Make sure to set up your NEXT_PUBLIC_POLICY_ID
in your environment variables.
Implement the Create Wallet Button
Create the button component that uses the configured provider.