Integrating with ecosystem wallet connectors
Integrating apps do not need to use Openfort themselves to integrate ecosystem wallets; instead, they can import a your ecosystem SDK directly to configure with their wallet connector library. Simply follow the instructions below to get set up!
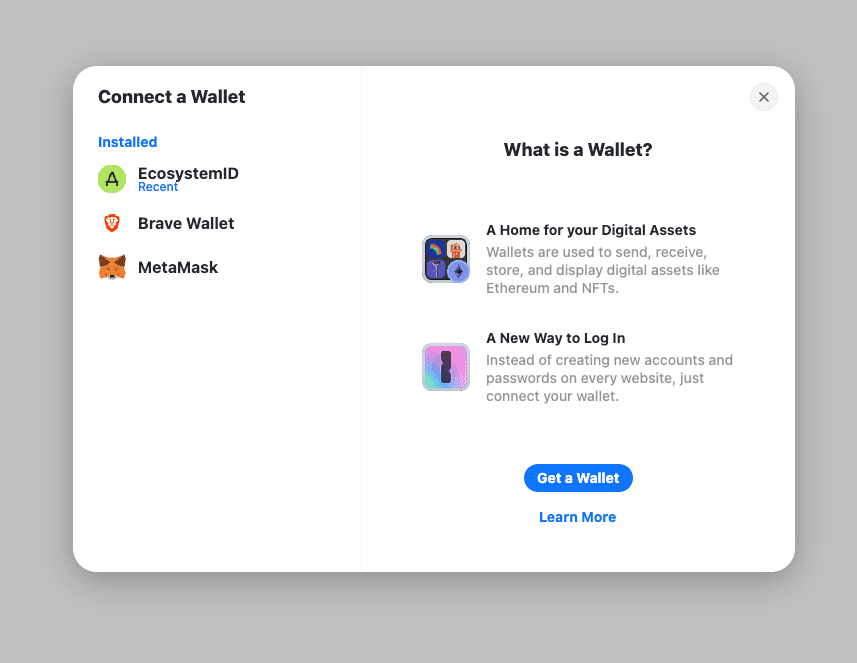
1. Install dependencies#
Install the @ecosystem/wallet
SDK and its peer dependencies:
To make these instructions concrete, this will continue with the sample ecosystem wallet called ... Ecosystem Wallet. For the sake of this guide, available in package form as @ecosystem/wallet
.
_10npm i @ecosystem/wallet @rainbow-me/rainbowkit @tanstack/react-query
2. Create the connector#
3. Wrap app with providers#
At the highest level of your applications, wrap the component with the wagmi, QueryClient, and RainbowKit providers. Pass the configuration you created in step 2 to the wagmi provider.
All of ecosystem wallets can export a standard EIP-1193 provider object. This allows your app to request signatures and transactions from the wallet, using familiar JSON-RPC requests like personal_sign
or eth_sendTransaction
.
EIP-1193, also known as the Ethereum JavaScript API, is a standardized interface for how applications can request information, signatures, and transactions from a connected wallet.
To get a wallet's EIP-1193 provider, use the getEthereumProvider
method:
4. Use the ConnectButton
#
Import the ConnectButton
and use to prompt users to connect to their provider ecosystem wallet.
_11import {ConnectButton} from '@rainbow-me/rainbowkit';_11_11function Page() {_11 return (_11 <div>_11 <h1> My app </h1>_11 ..._11 <ConnectButton />_11 </div>_11 );_11}
Thats it! You can now use any wagmi hook in your application to interact with the connected wallet. When users connect and transact with their wallet, Openfort will open a pop-up for users to authorize any actions.